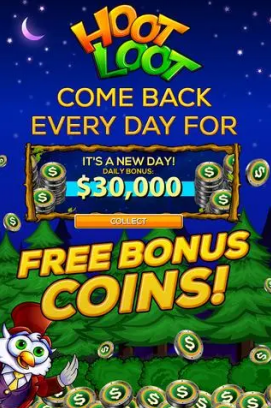
## Understanding Mongoose Login
In the ever-evolving world of web development, user authentication is a crucial aspect of any application. Mongoose, a popular Object Data Modeling (ODM) library for MongoDB and Node.js, simplifies this process significantly. This article will delve into the essentials of implementing a login system using Mongoose, outlining key concepts and steps involved.
### 1. What is Mongoose?
Mongoose provides a straightforward way to interact with MongoDB databases. It allows developers to define models and schema, ensuring that data structures are maintained consistently throughout the application. This structure is particularly beneficial when building robust login capabilities.
### 2. Setting Up Your Environment
**2.1 Installing Necessary Packages**
To get started, you'll need to install Node.js, MongoDB, and several npm packages:
```bash
npm install mongoose express bcryptjs jsonwebtoken dotenv
```
- **express**: A web framework for Node.js.
- **bcryptjs**: A library to hash passwords securely.
- **jsonwebtoken**: For creating and verifying JSON Web Tokens.
- **dotenv**: To manage environment variables safely.
**2.2 Connecting to MongoDB**
Once your packages are installed, establish a connection to your MongoDB database using Mongoose:
```javascript
const mongoose = require('mongoose');
mongoose.connect(process.env.MONGODB_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
```
### 3. Creating User Schema
Before users can log in, a User model must be defined. This model will include necessary fields such as email and password. Using Mongoose, define your user schema as follows:
```javascript
const userSchema = new mongoose.Schema({
email: { type: String, required: true, unique: true },
password: { type: String, required: true },
});
// Hash the password before saving it to the database
userSchema.pre('save', async function (next) {
if (!this.isModified('password')) return next();
this.password = await bcrypt.hash(this.password, 10);
next();
});
const User = mongoose.model('User', userSchema);
```
### 4. Implementing Registration
Creating a registration endpoint allows new users to sign up. Here’s how you can implement it:
```javascript
app.post('/register', async (req, res) => {
const { email, password } = req.body;
try {
const user = new User({ email, password });
await user.save();
res.status(201).send('User registered successfully');
} catch (error) {
res.status(400).send('Error registering user');
}
});
```
### 5. Implementing Login
The login functionality authenticates users by validating their credentials:
```javascript
app.post('/login', async (req, res) => {
const { email, password } = req.body;
const user = await User.findOne({ email });
if (!user) return res.status(401).send('Invalid credentials');
const isMatch = await bcrypt.compare(password, user.password);
if (!isMatch) return res.status(401).send('Invalid credentials');
const token = jwt.sign({ id: user._id }, process.env.JWT_SECRET, { expiresIn: '1h' });
res.status(200).json({ token });
});
```
### 6. Protecting Routes
To protect certain routes from unauthorized access, implement middleware that checks for valid tokens:
```javascript
const authMiddleware = (req, res, next) => {
const token = req.header('Authorization')?.split(' ')[1];
if (!token) return res.status(403).send('Access denied');
try {
const verified = jwt.verify(token, process.env.JWT_SECRET);
req.user = verified;
next();
} catch (error) {
res.status(400).send('Invalid token');
}
};
```
### Conclusion
With the steps outlined above, you should now have a fundamental understanding of setting up a login system using Mongoose. By implementing user registration, login functionality, and route protection, you've laid the foundation for securing user data in your application. As you develop further, consider researching more advanced techniques to enhance your authentication process, such as OAuth or multi-factor authentication. Happy coding!
**Word Count: 517 words**